Hey developers, Today I’m going to explain one of the confusing topic in Django. We’re going to discuss Django Models Relationship e.g OneToMany and ManyToMany. These are two basics relationship in Django database that are found quite confusing to beginners.
So, I’m going to explain both of these two relationship one by one with an Example.
Django Models Relationship
First, Let me show you my Models.py Code where I’ve shown both of these relationships among different models.
models.py
from django.db import models
# Category Model
class Category(models.Model):
name = models.CharField(max_length=20)
def __str__(self):
return self.name
# BlogPost Model
class Post(models.Model):
title = models.CharField(max_length=255)
body = models.TextField()
created_on = models.DateTimeField(auto_now_add=True)
last_modified = models.DateTimeField(auto_now=True)
categories = models.ManyToManyField('Category', related_name="posts")
def __str__(self):
return self.title
# Comment Model
class Comment(models.Model):
author = models.CharField(max_length=60)
body = models.TextField()
created_on = models.DateTimeField(auto_now_add=True)
post = models.ForeignKey('Post', on_delete=models.CASCADE)
In the above models.py, I’ve created different models for A Fully Functional blog. Where you could see three different models e.g Post, Category and Comment. Now Let’s start our Django Models Relationship Guide.
So, First we will talk about ManyToMany Django Database Realationship.
Django ManyToMany Model Relationship:
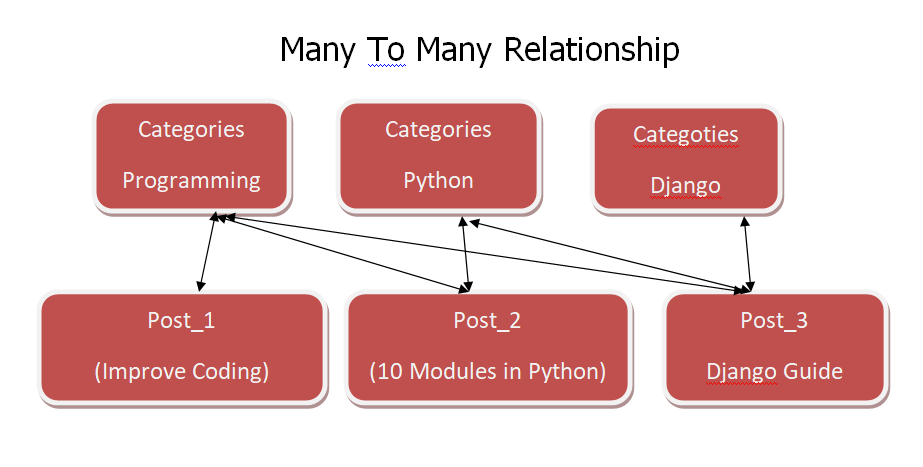
In this ManyToMany Relationship, We can have models that could be related to more than one posts. This models will be not lies under any particular models.
Example:
Let’s Suppose we’ve created 3 Post With the Title as given below:
- Post_1: How To Improve Coding Skills
- Post_2: Top 10 Modules in Python
- Post_3: Django Full Guide For Beginner.
Now, We have also 3 different Categories from Category Modules:
- Programming
- Python
- Django
Now, here you could see that Post_1 will belongs to only 1 categories(Programming). Where Post_2 will be also belongs to First two categories(Programming, Python) and Finally Post_3 will have all these categories(Programming, Python and Django).
Here, You can see that either a post or categories is not just limited to single post or categories. Which means In ManyToMany Django Relationship, “A single Post can have different categories and as same as A categories could be belongs to different posts”
In the above “models.py“, You can see in the Post Model that I’ve defined categories by using ManyToMany field. You can define ManyToMany field by using following code:
categories = models.ManyToManyField(‘Category’, related_name=”posts”)
Here, I’ve filled ‘Category’ Model in place of class that I want related with the Post Model.
You May Also Like: How To Add Bootstrap 4 Form in Django with crispy-forms
Django OneToMany Model Relationship:
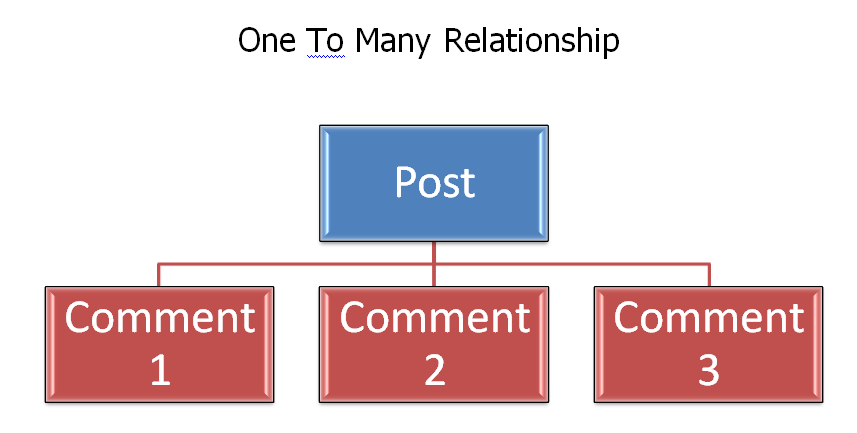
In this this Relationship An Object of a model will be related to only Single Model Object. Which means Like A model can have different objects from other models. But A Child model couldn’t belongs to other Model. That Child model will be related to only a single model.
For Example:
In ManyToMany, Post could have different categories and A categories could belongs to different Post Model.
But Here, A Post Model can have different Comments like, Comment_1, Comment_2, Comment_3. But Here, these comments will be not related to other posts like Post_2 and Etc. As a result whenever someone would be delete Post_1, then all these comments related to Post_1 would be deleted.
It’s also help to design database for specific users, So that each user will have their own database to store information.
In the above Models.py code, I’ve defined that how you could make OneToMany Relationship among Models.
Here, You’ll need to use Foreign Key field into main Child Class Model to connect it with Parent class model.
post = models.ForeignKey('Post', on_delete=models.CASCADE)
You need to add above code in Comment model. After than any created comment would be belongs to only specific Post Model Object.
Here, You can see that I’ve filled two parameters – First I filled ‘Post’ that refers comment would be related to only one Post. Second we have on_delete=models.CASCADE, Which means whenever you will delete any Post then all the comments related to that specific Post will be automatically delete.
Short Explanation:
OneToMany Relationship: In this relationship, A model can have different Child models but all those child model will be related to only this particular model.
ManyToMany Relationship: In this relationship, Both of the model could be related to each others. Example: Post And Comments.
I hope you all will be like this article. Please don’t forget to share this article with your friends and geeks. You can also bookmark or follow our blog for such kind of programming updates.
Thank’s To Visit…