Arrow function in Javascript is one of the most useful feature that was introduced in JavaScript EcmaScript 6. You can also consider it as an anonymous or one line function. It also works differently than traditional functions when it comes to handling this keyword.
In this article, I’m gonna give you brief explanation about what is arrow function and When you should use it.
Arrow function Vs Normal Function
First, let’s compare what are the syntactical difference between Arrow function and Normal function. For example, I’m going to write a simple code.
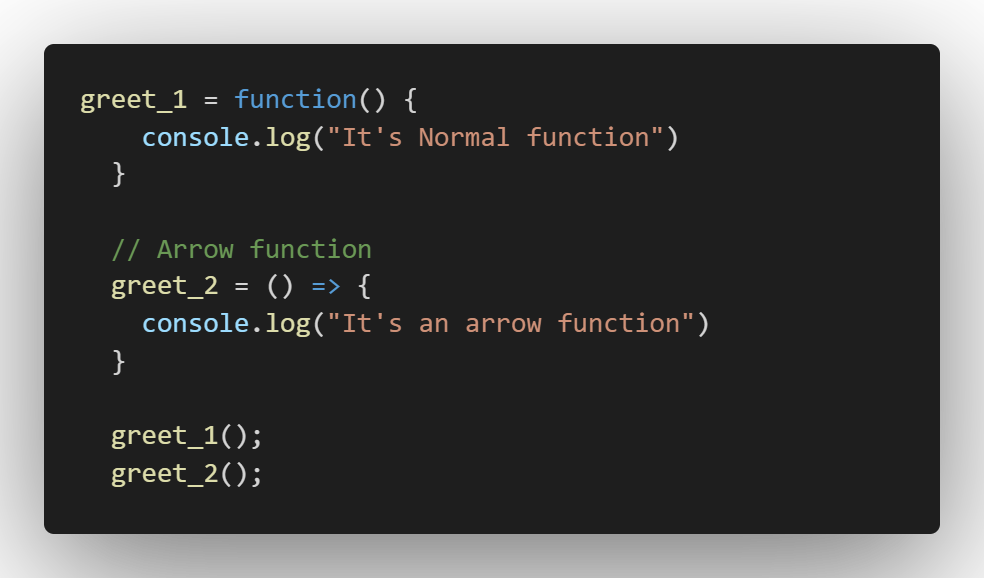
Basically, we define arrow function with () => { }, we don’t need function keyword here to define it. And if you have one line of function which returns something, then in such situation arrow function is most concise and short way to define same expression….
// with traditional method
function add_1(a,b) {
return a + b
}
// WIth arrow function
add_2 = (a, b) => a + b
console.log(add_1(2,3))
console.log(add_2(2,3))
In the above code, you can clearly see that by using the arrow function we can write the same code in one line. All you need to pass arguments and then return the expression you want. (args…) => { expression }
Arrow function as an Argument:
It also makes your code much clearer when you want to use a function as an anonymous function without declaring it.
So, we all know that the map function takes one function as an argument to pass elements of objects through it in each iteration. Here we can use the arrow function instead of the normal function to make our code much cleaner.
yearOfbirths = [1999,2001,2002,1998,1992]
// With Normal Function
ages_normal = yearOfbirths.map(function(el){
return 2021 - el;
})
// With arrow function
ages_arrow = yearOfbirths.map(el => 2021 - el);
console.log(ages_normal)
console.log(ages_arrow)
In the above code, you can see that In the map method, where we used the arrow function is much cleaner. All we declared was argument(el) and then expression (2021 – el).
Read also: Map function in python(BASIC)
Lexical “this” keyword in arrow function:
using “this” keyword inside an arrow is different than using it inside normal function.
So, basically arrow function uses “this” keyword from its surrounding, whereas normal function don’t use “this” keyword in such way.
We all know that “this” keyword point to object when your work with method. But when you use regular functions then this keyword points to a global object. While in ES6 arrow function gathers this keyword refers to their surrounding scope, not to global object.
That’s it for arrow function. So, I hope you liked this article, If yes then please don’t forget to share this article with your friends. You can also subscribe our blog via email to get future notification from our blog.
Thanks to read…
1 thought on “Arrow Function in JavaScript ES6 (GUIDE)”