Hey coders, today we are going to see basic string manipulation and methods in python programming language.
What is String?
In python, a string is a sequence of characters, where they are surrounded by double quotes(” “, or ‘ ‘). Anything between these quotes will be considered as a string, e.g: “My name is pk karn“.
Now there are lot things that you can do with these strings, e.g. string manipulation, operations. And there are also some inbuilt method to perform specific operation on string.
In python, strings are also considered as an Arrays, thus you can access characters at specific position using index value.
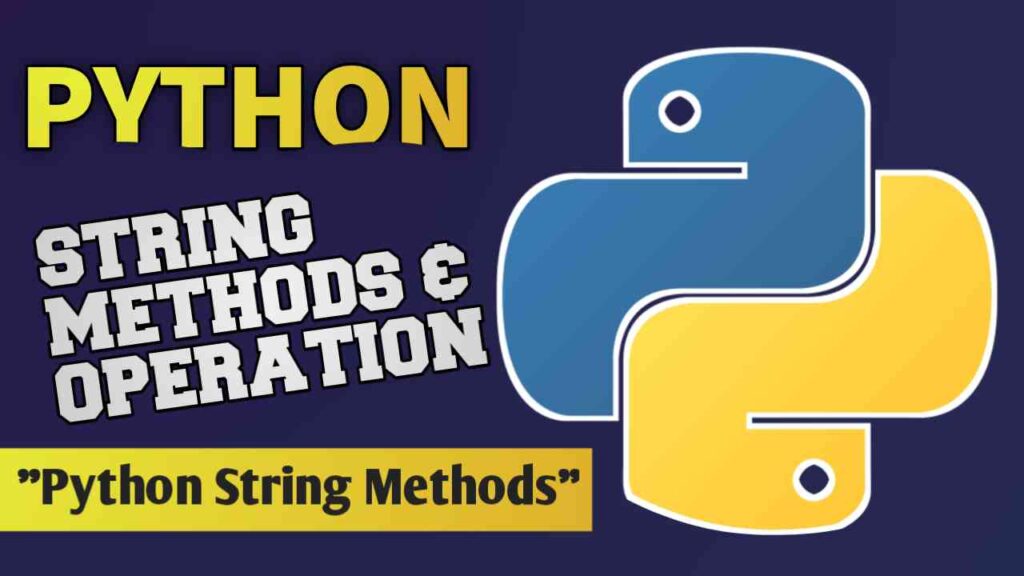
Now let’s know more about string.
Multiline Strings:
To write multiline strings, you can use triple quotes like:
a = """I'm PK Karn,
and I love coding and blogging,
that's why I'm sharing this knowledge with you."""
Strings as Array & Slicing:
In python, strings are like an array, thus you can access any characters from any position. For example, if you want to access the character of 1st position then you need to put index 0 inside []
a = "Python is programming language"
print(a[0])
#Output: P
#------------------
a = "Python is programming language"
print(a[6:]) # It will give you output from position of 6th character.
#Output: is programming language
a = "Python is programming language"
print(a[10:22]) # To access element between specific range
#output: programming
Looping through string:
You can also loop through a string using for loop, with help of this you can iterate each character of string e.g:
for x in 'programmer':
print(x)
:Output
p
r
o
g
r
a
m
m
e
r
To calculate String length:
You can use len() function to calculate length of strings, e.g:
name = "pkkarn"
print(len(name))
#output: 6
To Check characters or phrase in a string:
You can check whether a specific character or phrase exists in a string or not, by using in keyword. If it would exist then it will return a boolean result e.g. True
name = "pk karn"
if "pk" in name:
print("It exists")
# output: It exists
String Methods
Now, let’s see some string methods that you can use to perform some specific action on a string. I’m gonna show you some important string methods that you will be using more often.
capitalize(): To convert first char to uppercase:
capitalize() method converts first character to uppercase e.g “My name is pk karn”
name = "my name is pk karn"
print(name.capitalize())
# Outpup: My name is pk karn
casefold(): To convert entire string to small character:
name = "PROGRAMMER"
print(name.casefold())
# Outpup: programmer
find() and index(): To find index position value:
we can use find() and index() both to find position of specified value from string.
text = "my name is pk karn."
x = text.find("name")
print(x)
format(): to insert a value of a variable inside string:
You can use fromat() method to insert value of variable inside a particular string, :
formatMoney = "$ {price}"
print(formatMoney.format(price = 200))
#output :$ 200
These are the basic of string in pyhton, you can read lot more about python string from their official docs.
Read also: Exception Handling in Python(Guide)
I think you liked this article, if yes then please don’t forget to share with other programmers. You can also subscribe to our blog to get such kinds of articles from us.
Thanks to visit…
1 thought on “Python Basic: String Methods and Operations (Basic Guide)”